Adventures in the Wobbly Universe of CWE-787: A Buffer Overflow Odyssey
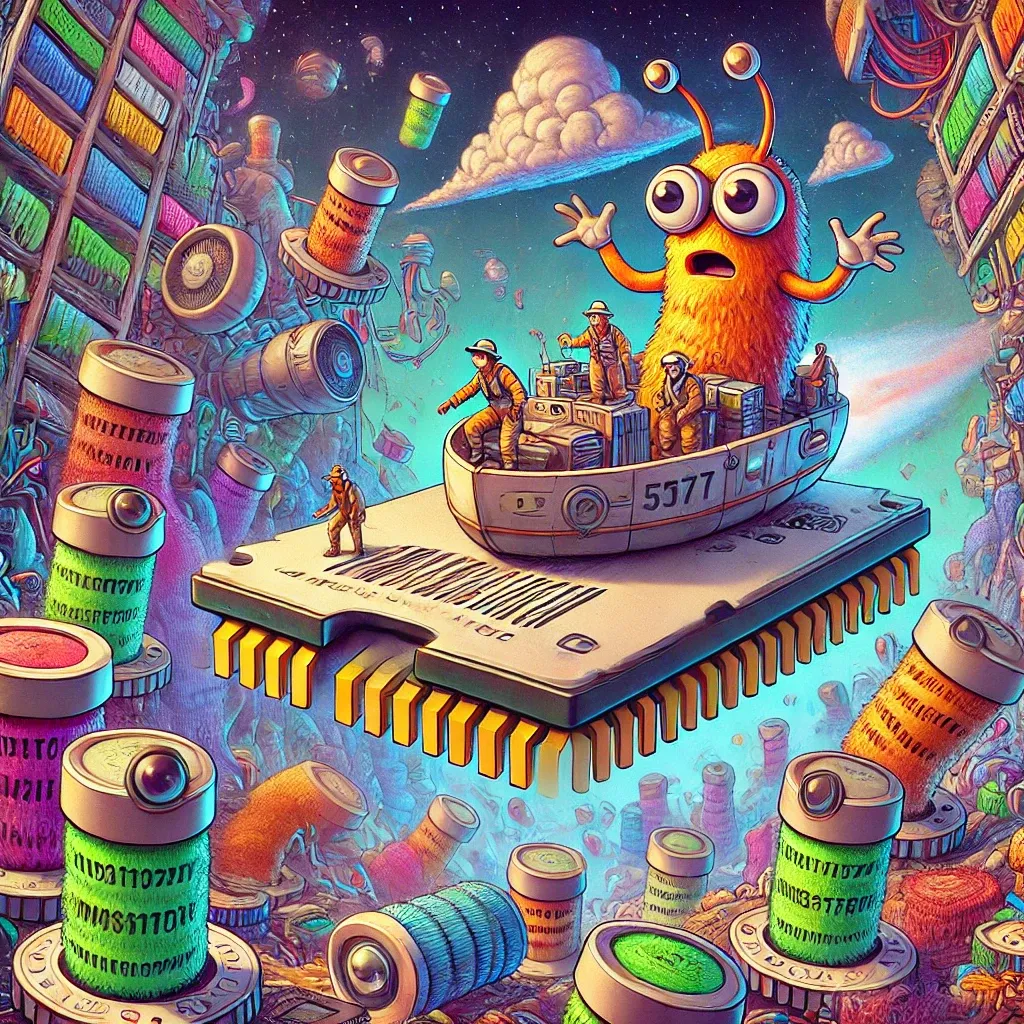
Let me take you on a journey into the perilous world of computer vulnerabilities, a place that makes navigating through airline baggage fees seem downright trivial. Today's thrilling escapade is into the heart-stopping, data-mangling drama of CWE-787: the "Out-of-Bounds Write."
Ah, the buffer overflow—a concept that strikes fear into the hearts of software developers, thrills the mischief-loving hacker, and leaves the rest of us squinting at our screens wondering why the computer suddenly decided to reboot itself into oblivion. But what exactly is CWE-787, and why does it sound like an airline route to disaster? Let’s unpack this digital Pandora's box together.
The Buffet Table of Buffers
Picture this: you're at an all-you-can-eat buffet. The sign says, "Please fill only your plate." But someone—let's call him Chad—piles mashed potatoes not only on his plate but also on the table, the chairs, and (somehow) the poor guy’s shirt in the next seat. This, in a nutshell, is what happens during a buffer overflow. A program tries to stuff more data into a memory area (the "plate") than it was designed to hold, and soon, there’s mashed potato chaos everywhere.
Now, when Chad does it, he gets a stern talking-to from management. When your software does it, things go haywire: systems crash, data corrupts, and in some cases, malicious folks use the chaos to slip in some of their own nasty surprises. Fun, right?
A Quick Crash Course in Out-of-Bounds Writes
CWE-787, for those uninitiated in the taxonomy of software horrors, stands for "Common Weakness Enumeration 787." It’s a posh way of saying, "Your program just scribbled outside the lines and broke something." This happens when code writes data to an address it shouldn't, often because it’s gone merrily traipsing past the end of an allocated memory buffer.
Imagine writing a letter to a friend and, running out of space on the paper, deciding to continue your heartfelt prose on their dining table, their cat, and their neighbor’s garage door. Except instead of awkward apologies and white paint, the result is your system face-planting or—if you're very unlucky—handing control of your computer to a hacker who finds your creative expression delightful.
Example 1: The Classic C Mistake
#include <string.h>
#include <stdio.h>
int main() {
char buffer[10];
strcpy(buffer, "This string is way too long for the buffer!");
printf("%s\n", buffer);
return 0;
}
In this example, strcpy
doesn’t check if the source string fits into the destination buffer. The result? Data spills over the bounds of buffer
, corrupting nearby memory. The program might crash or behave unpredictably.
Example 2: A Hacker’s Playground
#include <stdio.h>
#include <string.h>
void vulnerableFunction(char *input) {
char buffer[20];
strcpy(buffer, input);
}
int main() {
char maliciousInput[40] = "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBBBCCCCDDDD";
vulnerableFunction(maliciousInput);
return 0;
}
Here, a carefully crafted input overwrites the buffer and potentially alters the return address of the function, allowing an attacker to execute arbitrary code.
System Crashes: The Software's Dramatic Exit
When a buffer overflow occurs, your system often has no idea what to do. It’s like accidentally hitting the power button on a rollercoaster mid-ride. Programs might freeze, terminate abruptly, or descend into an error message so cryptic you’d swear it’s an ancient prophecy. And when the overflow starts overwriting critical system data, the entire operating system can grind to a halt, leaving you with nothing but a black screen and a deep sense of existential despair.
Code Execution: A Hacker’s Playground
Worse still, out-of-bounds writes open the door for a bit of hacker mischief called "arbitrary code execution." Here’s how it works: when an overflow overwrites memory, attackers can cleverly plant their own instructions into your program. Suddenly, your computer isn’t just crashing—it’s participating in a botnet, mining cryptocurrency, or sending spam about dubious pharmaceutical products to everyone in your contact list.
It’s like inviting someone to your dinner party, only for them to take over your kitchen and start hosting a rave for strangers while you watch helplessly from the pantry.
Preventing the Madness
So, how do you avoid this digital descent into madness? Here are a few handy tips:
- Bound Checking is Your Friend: Always validate inputs to ensure they’re within expected limits. Think of it as checking IDs at the door of your memory club—no gatecrashers allowed.
- Use Safer Functions: If you’re working in languages like C or C++, opt for safer alternatives (e.g.,
strncpy
overstrcpy
) and modern libraries that include bounds-checking. - Embrace Modern Tools: Static analyzers and dynamic testing tools can sniff out buffer overflow vulnerabilities before they hit production. It’s like having a guard dog for your code—except it doesn’t shed.
- Avoid Playing with Sharp Objects: If you’re not a seasoned programmer, consider using languages like Python or Java that handle memory for you. Sure, they’re not perfect, but they’re less likely to stab themselves in the leg with a pointer.
- Regular Updates: Keep software, libraries, and dependencies up to date. Developers regularly patch vulnerabilities, so running an old version is like leaving your doors unlocked with a sign saying, "Please rob me."
Final Thoughts
In the grand symphony of computing, CWE-787 is the rogue violinist who’s not only out of tune but also hurling bows at the conductor. It’s a reminder that software, like life, is fragile and prone to chaos when boundaries aren’t respected.
So, next time your computer crashes unexpectedly or some hacker uses your email to peddle suspicious herbal supplements, spare a thought for the humble buffer—and the chaos it unleashes when it forgets its place. And remember: always check your bounds, or your mashed potatoes might just end up on someone else’s table
Comments ()